Table of Contents
Object Oriented Programming Language (OOPs)
Introduction
Programs written in high level languages like COBOL FORTAN or C are called procedure oriented programs. In this type of programming, the tasks to be performed by the program are seen as a sequence. More than one function is written to perform these tasks. But while focusing on the development of functions, we forget the data that is to be used by these functions. In general, the merits (demerits) of procedure oriented programs can be determined in this way.
- Programs are based on algorithms.
- Programs are divided into smaller programs/functions.
- Universal data is used i.e. the same data is used by many programs.
- Data can move freely from one function to another.
- Top-down concept is used for program design.
Object Oriented Programming has been developed to find solutions to the above problems. In object oriented programming, also called Object Oriented Programming in short, data is considered an important part of program development instead of functions. In this, data is associated only with those functions that are to use it. In this way, data remains protected from use in external functions and possible changes that may occur by them. The structure of data and functions in an object oriented program is shown in the following figure.
Based on this diagram, the definition of object oriented programming can be given as follows: “Object oriented programming is a concept that modularizes a program by creating separate memory areas for data and functions in such a way that they can be used like paperbacks for copying modules.”
Basic Concept of OOPs:
- C++ is an object oriented programming language.
- C++ developed by Bjarne Stroustrup at AT & T Bell Lab. In Murray Hill, New Jersey, USA in early 80s.
- C++ is super set of C.
- It support object oriented programming features and still retain the power and elegance of C.
- Every C++ program must have a main ( ). C++ is a free form language.
An object-oriented program may have the following characteristics:
- It focuses on data rather than procedures.
- The program is divided into objects.
- Data structures are designed in such a way that they reflect objects.
- Data is tied to the functions that use it in such a way that it cannot be used by other external functions.
- New data and functions can be added at any time.
- The bottom-up concept is used in programming.
General Concepts:
- Objects
- Classes
- Data Abstraction
- Data Encapsulation
- Inheritance
- Polymorphism
- Message Passing
- Dynamic Binding
Classes and objects:-
- Object model is defined by means of classes and objects.
- Oriented programming languages are based on object oriented technology. Example: book, pen, student, employee, machine etc.
- Class is a data type, which support data abstraction.
- Class is a prototype or blue print or model that defines different features.
- A feature may be a data or an operation.
- Data are represented by data member or instance variables.
- Operations are also known as behaviors or methods or functions.
- Object is an instance of a class.
Advantages of classes are
- Modularity
- Information hiding
- Reusability
Mango is a fruit: fruit is a class and mango is an object of fruit class.
Difference between class and object
S. | Class | Object |
1 | Class is a data type | Object is an instance of class data type |
2 | It generals object | It gives life to a class |
3 | It is the prototype or model | It is a container for storing it’s features |
4 | It does not occupy memory location | It occupies memory location |
5 | It is cannot be manipulator because it is not available in memory | It can be manipulated |
Data Abstraction
- Abstraction refers to the act of representing essential features without including the background details or explanations.
- Abstraction explains ‘what’ and ignores ‘How’.
- Class defined as a list of abstract attributes such as – Size , Weight and Cost and functions to operate on these attributes.
- Encapsulate all the essential properties of the objects that are too created.
- Classes use the concept of abstraction and therefore they are known as Abstract Data Type (ADT).
- Abstraction separates interface and implementation.
Data Hiding or Encapsulation:-
- The wrapping up of data function into a single unit (called class) is known as encapsulation.
- This encapsulation of the data from direct access by the program is called data hinding.
- The term encapsulation is used to describe the hiding of the implementation details.
- The advantages of encapsulation are
- Information hiding
- Implementation Independence
Inheritance
- C++ strongly supports the concept of reusability.
- The C++ classes can be re used in several ways. Once a class has been written and tested, it can be adapted by other programmers to suit their requirement. This is basically done by creating new classes, reusing the properties of the existing ones.
- Reusability not only save time and money but also reduce frustration and increase reliability.
- The mechanism of deriving a new class from an old are is called inheritance or derivation. The old class is referred to as the base class and the new one is called the derived class.
- A class also inherits properties from more than one class or from more than one level.
- When a member function / data members in the base class can be used by objects of the derived class. This is called accessibility.
Polymorphism
- One thing with several distinct forms is called polymorphism.
- It is oops concept.
- Polymorphism allows the same function to act differently in different classes.
- Polymorphism plays an important role in allowing objects having different internal structures to share the same external interface.
Ex.:
(a) Two number add by + operator given sum
X=5+9
(b) If two operands are string then result will concatenates two strings.
Str =”hello”+”bca”
Output
Hello bca
Dynamic Binding:-
- Binding refers to the linking of a procedure call to the code to be executed in response to the call.
- Binding means cannot one program to another program that is to be executed in reply to the call.
- Dynamic binding means that the code associated with a give procedure call is not known until the time of the call at runtime.
- It is associated with polymorphism and inheritance.
- In C++, dynamic binding achieve by virtual function.
- Virtual function defined in base class.
- To achieve dynamic binding in C++, create a pointer of base class.
Message Passing:-
- In oops object may communicate each other.
- Objects communicate each other much same way as people pass messages to one another.
- A message for an object is a request for execution of a procedure and therefore, will invoke a function in the receiving object that generates the designed result message.
- Object have a life cycle, they can be create and destroyed.
- Communication with an object feasible as long as it alive.
OOPs is better than traditional procedural Programming:
S | Procedural oriented | OOPs |
1 | Top down approach is followed. | Bottom up approach is followed. |
2 | Focus is an algorithm and control flow | Focus is an object model. |
3 | Program is divided into a number of function or procedure. | Program is organized by having a number of classes and object. |
4 | Functions are independent of each other. | Each class is related in a hierarchical manner. |
5 | View data and function as two separate entities. | View data and function as single entity. |
6 | Algorithm is given importance. | Data is given importance. |
7 | Function call is used. | Message passing is used. |
8 | Software reuse is not possible. | Help in software reuse. |
9 | No encapsulation data and function are spare. | Encapsulation package code and data algorithm. |
10 | Function abstraction is used. | Data abstraction is used. |
You May Like to Browers More
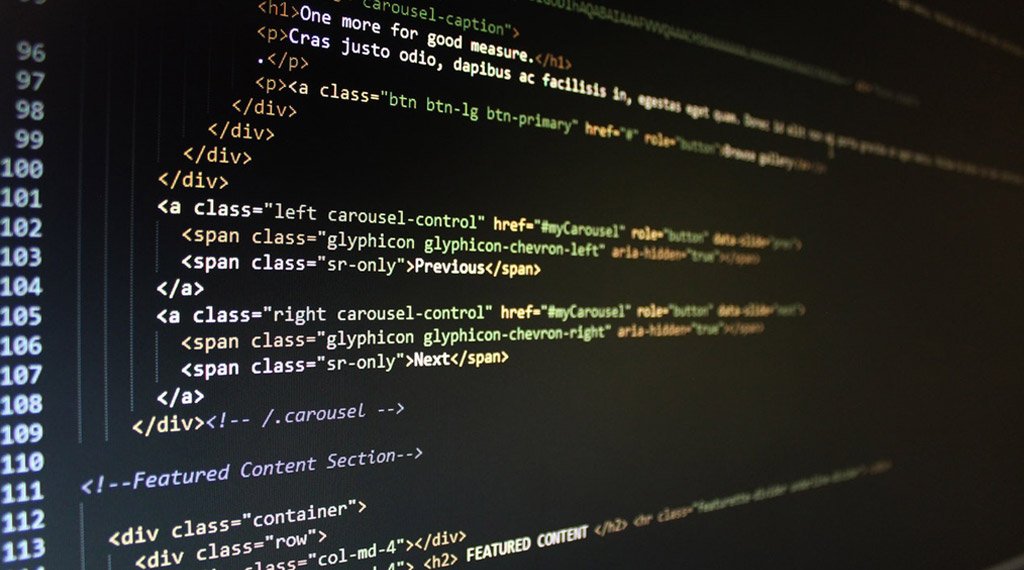
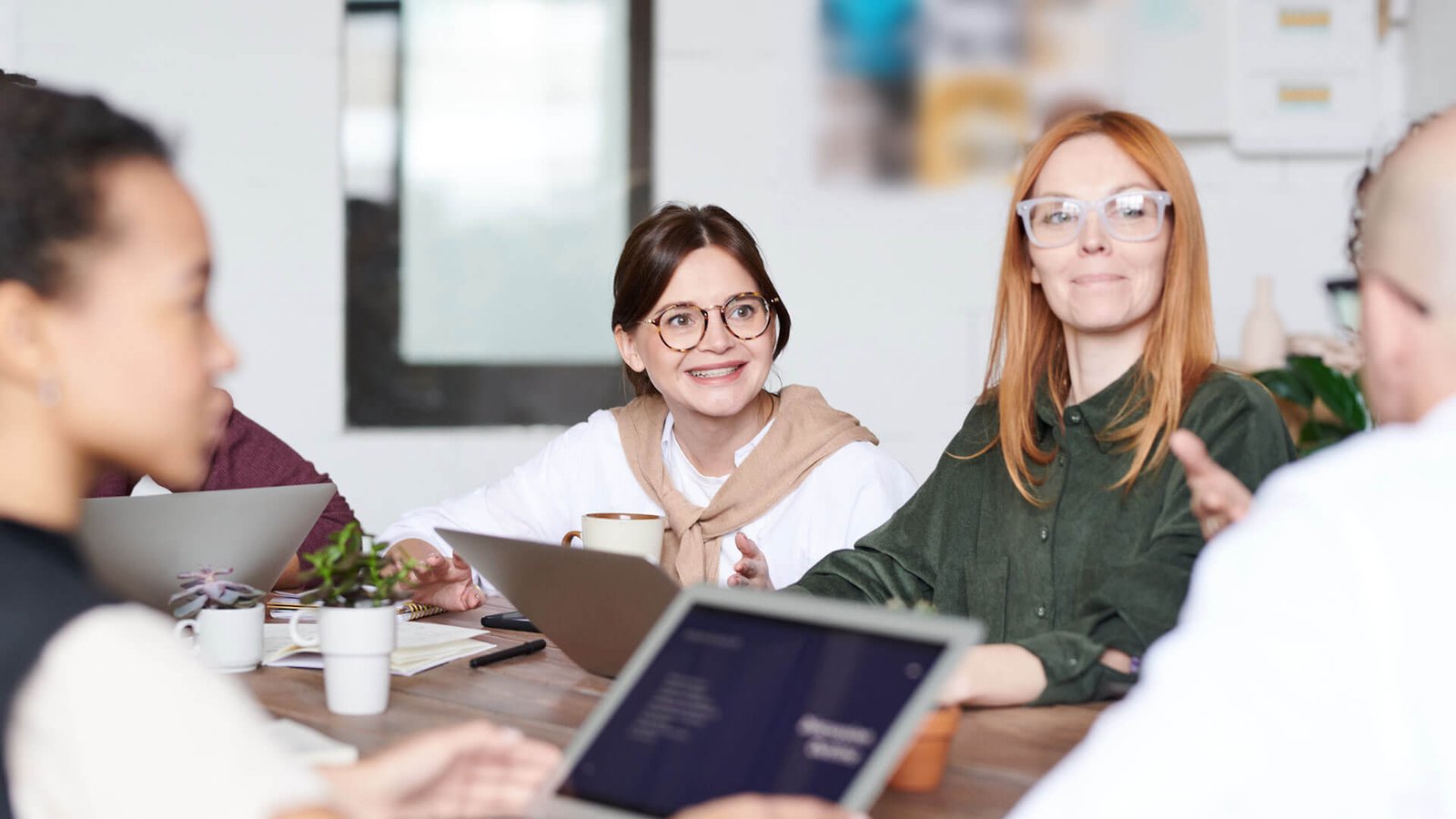
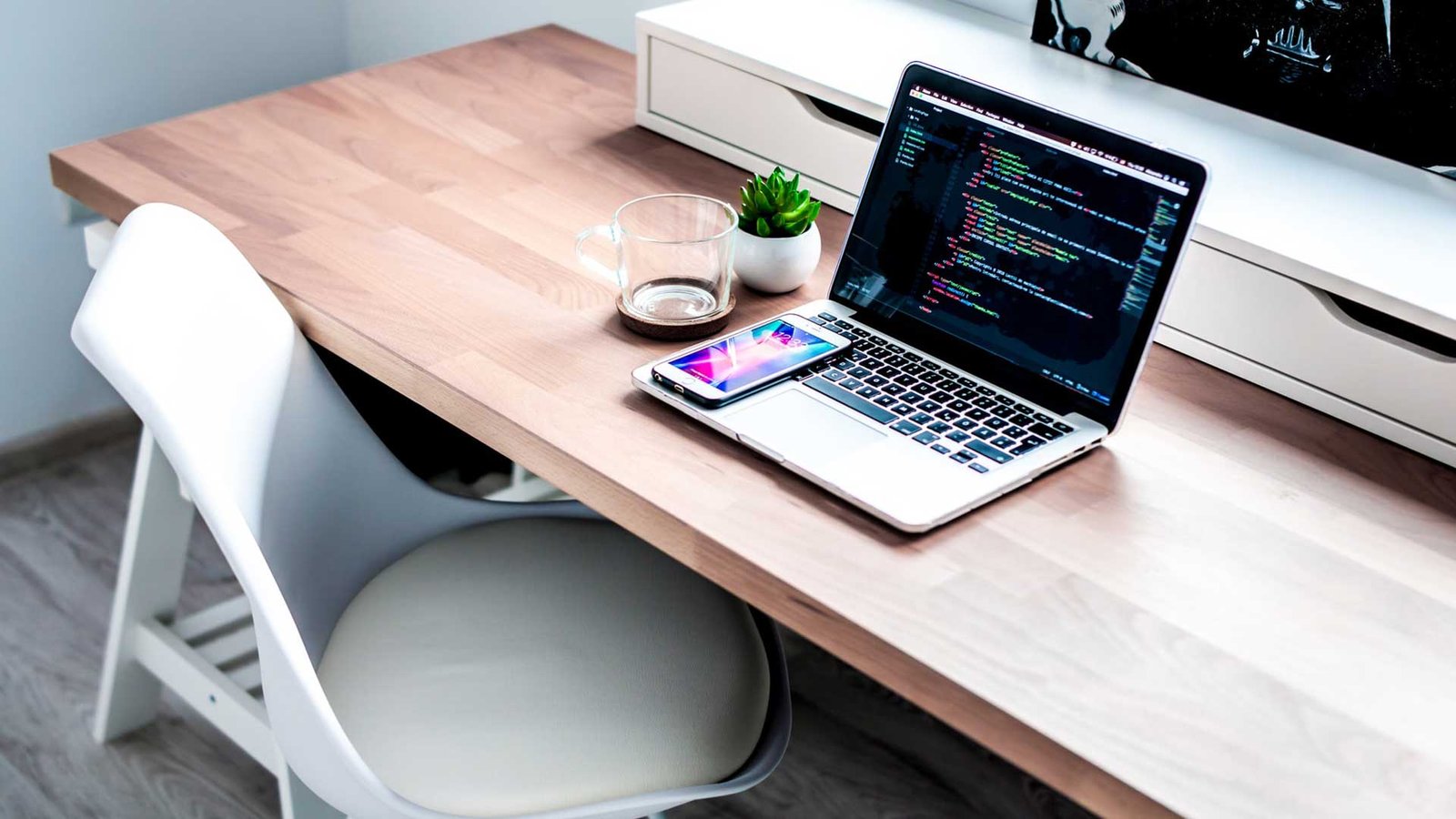