Table of Contents
C++ Static Data Member
- A data member of a class can be qualified as static. It has certain special characteristics.
- It is initialized to zero when the first object is created.
- Only one copy of that member is created for the entire class and is shared by all the objects of that class.
- It is visible only within the class, but its lifetime is the entire program.
- Static variable are normally used to maintain values common to the entire class.
- The type and scope of each static member variable must be defined outside the class definition. This is necessary because the static members are stored separately rather than as a part of an object.
- They are associated with the class it self rather than with any class object, they are also known as class variable.
Example:
#include <iostream.h>
#include<conio.h>
class student
{
private: static int code; //data member
int rollno;
public: static void init (int c) //data member function
{
code=c;
}
void getdata (int r)
{
rollno =r;
}
void show()
{
cout<<“Rollno = “<<rollno<<endl;
cout<<“Code = “<<code<<endl;
}
};
int student :: code;
void main ()
{
clrscr();
student :: init (108);
student obj1,obj2;
obj1.getdata(448);
obj2.getdata(224);
obj1.show();
obj2.show();
getch();
}
Output :
Rollno = 448
Code = 108
Rollno = 224
Code = 108
You May Like to Browers More
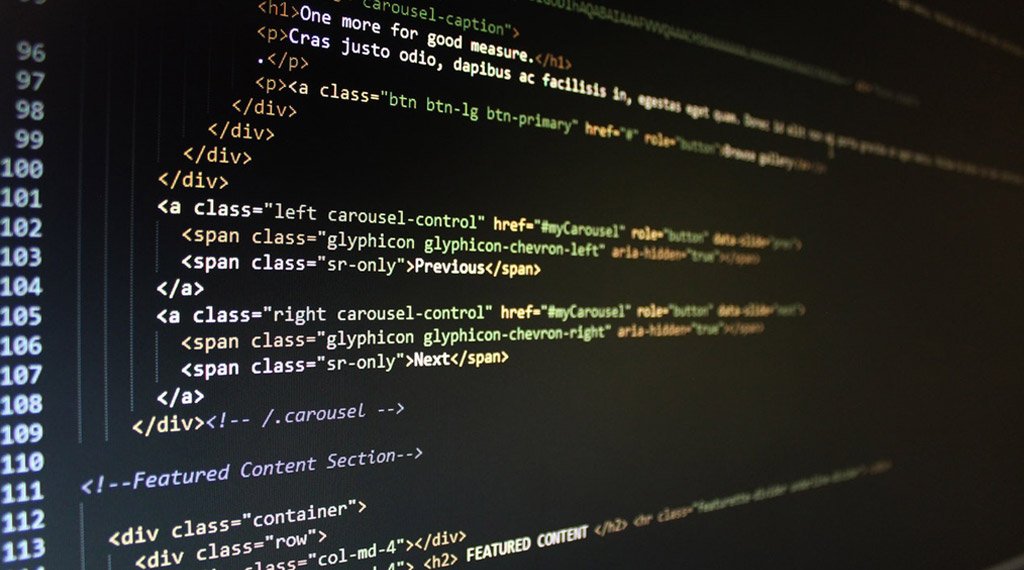
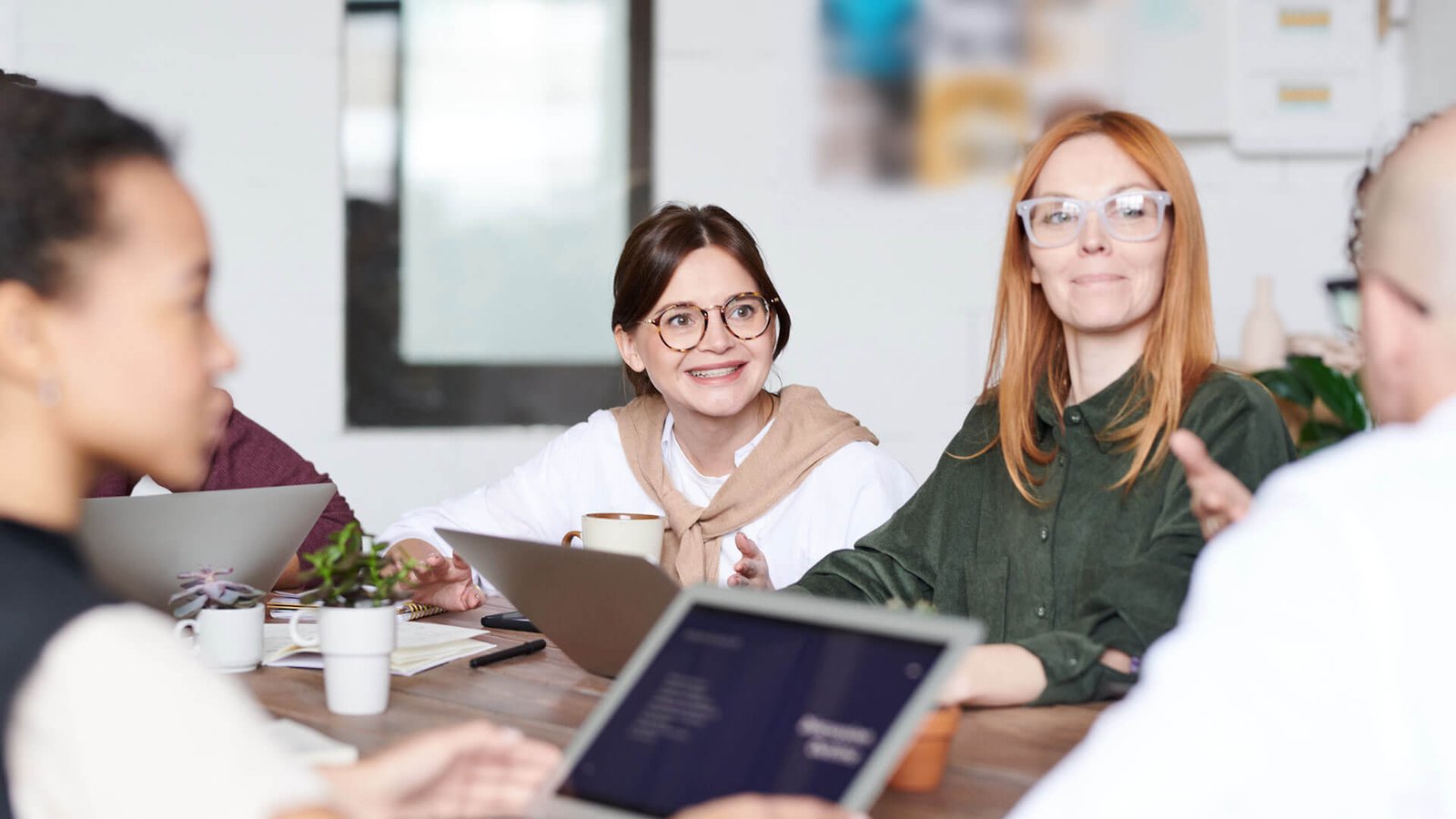
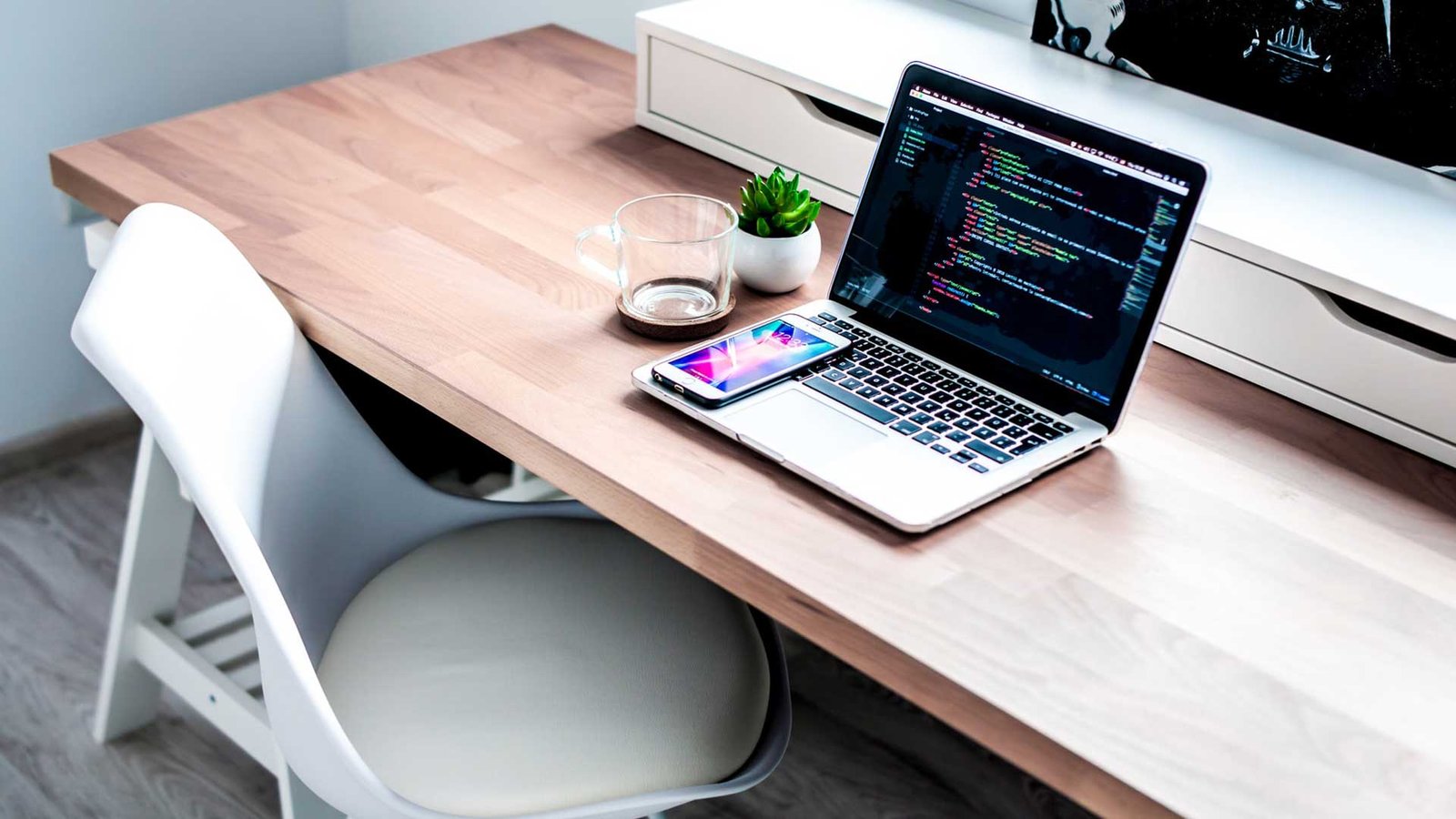