Table of Contents
Linked Stack and Queue in Data Structure
Stack and Queue as Linked List
Linked Stack:
Algorithm: PUSH (TOP, INFO, LINK, TEMP, ITEM)
This procedure adds new node TEMP to the stack.
Step 1: Allocate memory for new node TEMP.
Step 2: [ Store the ITEM into INFO part of new node ]
TEMP → INFO := ITEM
Step 3: [ Make the TEMP node points to TOP. ]
TEMP → LINK := TOP
Step 4: [ Reset TOP pointer. ]
TOP := TEMP.
Step 5: [ Exit ]
Return.
Algorithm: POP (TOP, INFO, LINK, TEMP, ITEM)
This procedure deletes TOP node from STACK and store the data of INFO part into ITEM variable.
Step 1: [Check whether STACK is empty or not. ]
If TOP = NULL then
Write (‘Underflow! Stack is empty’)
and return.
[ End of If structure ]
Step 2: [ Store the data of TOP node into ITEM. ]
ITEM := TOP → INFO
Step 5: [ Remove the TOP node ]
TEMP := TOP
TOP := TOP → LINK
Step 6: Free the TEMP node.
Step 7: [ Exit ]
Return.
Linked Queue:
Algorithm: ADD (INFO, LINK, FRONT, REAR, TEMP, ITEM)
This procedure adds new node TEMP to the QUEUE.
Step 1: Allocate memory for new node TEMP.
Step 2: [ Store the ITEM into INFO part of new node ]
TEMP → INFO := ITEM
Step 3: [ Make the TEMP node points to NULL. ]
TEMP → LINK := NULL
Step 4: [ Add the ]
Front:= TEMP.
Step 5: [ Exit ]
Return.
Algorithm: POP (TOP, INFO, LINK, TEMP, ITEM)
This procedure deletes TOP node from STACK and store the data of INFO part into ITEM variable.
Step 1: [Check whether STACK is empty or not. ]
If TOP = NULL then
Write (‘Underflow! Stack is empty’) and return. [ End of If structure ]
Step 2: [ Store the data of TOP node into ITEM. ]
ITEM := TOP → INFO
Step 5: [ Remove the TOP node ]
TEMP := TOP
TOP := TOP → LINK
Step 6: Free the TEMP node.
Step 7: [ Exit ]
Return
You May Like to Browers More
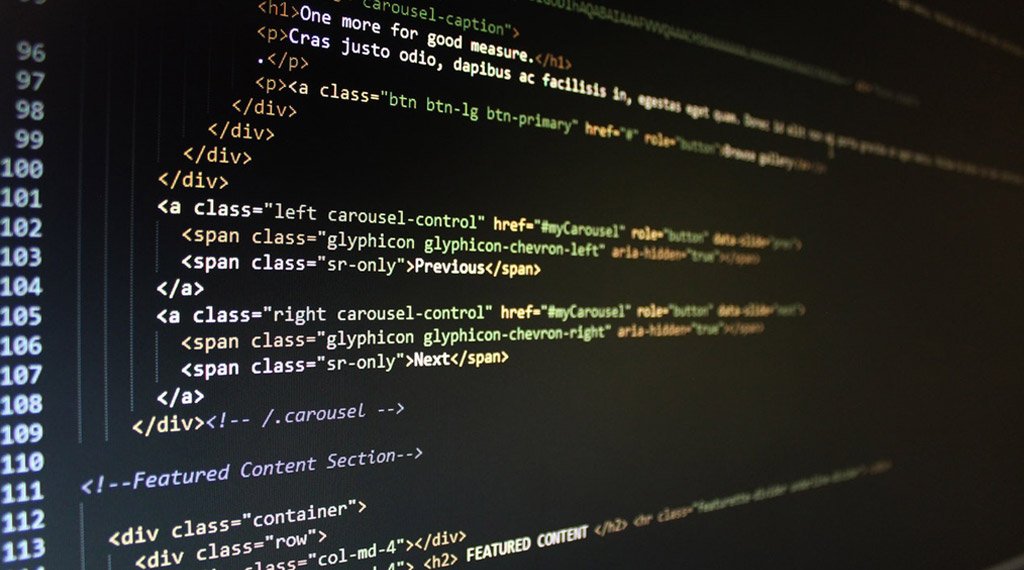
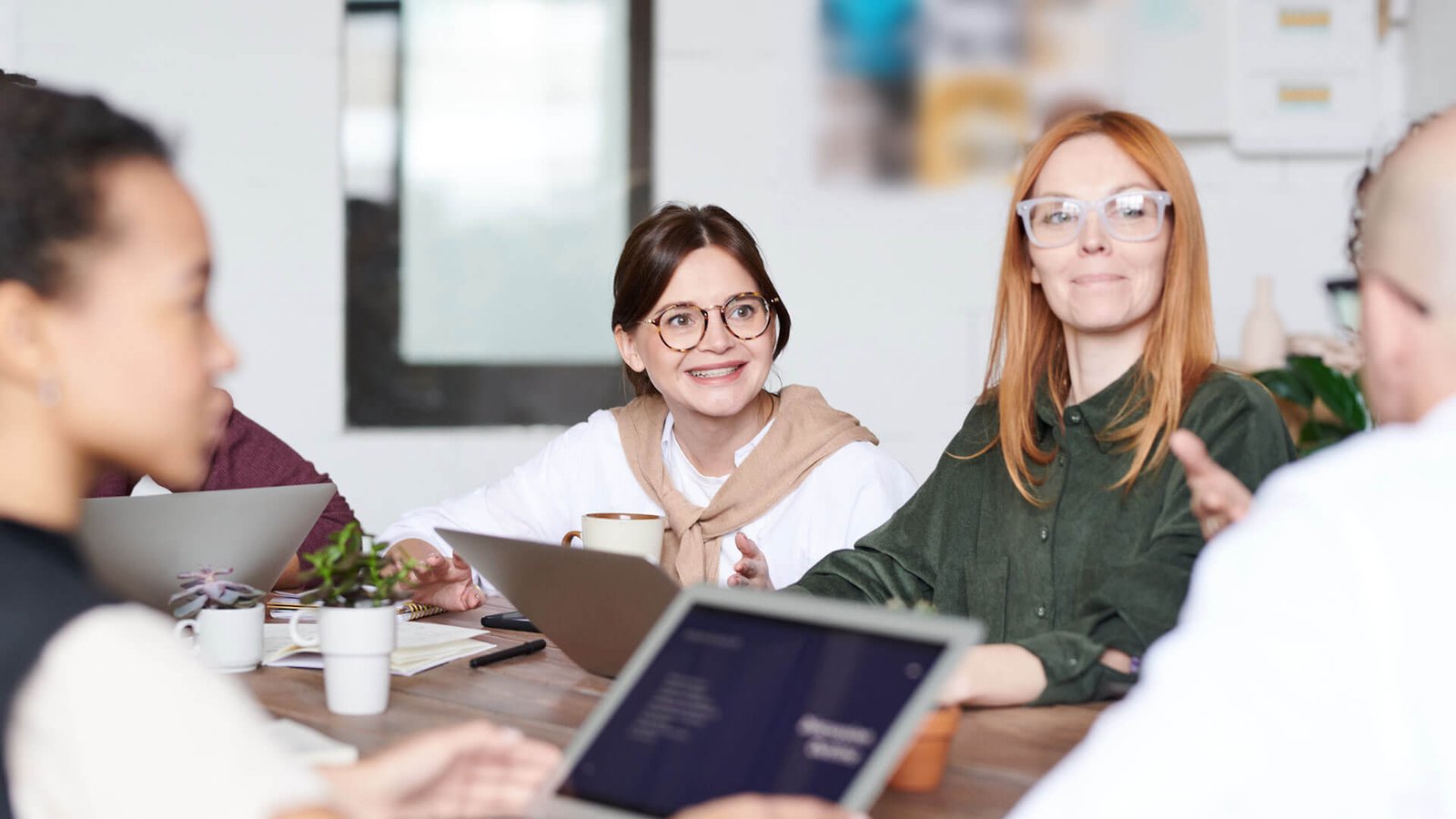
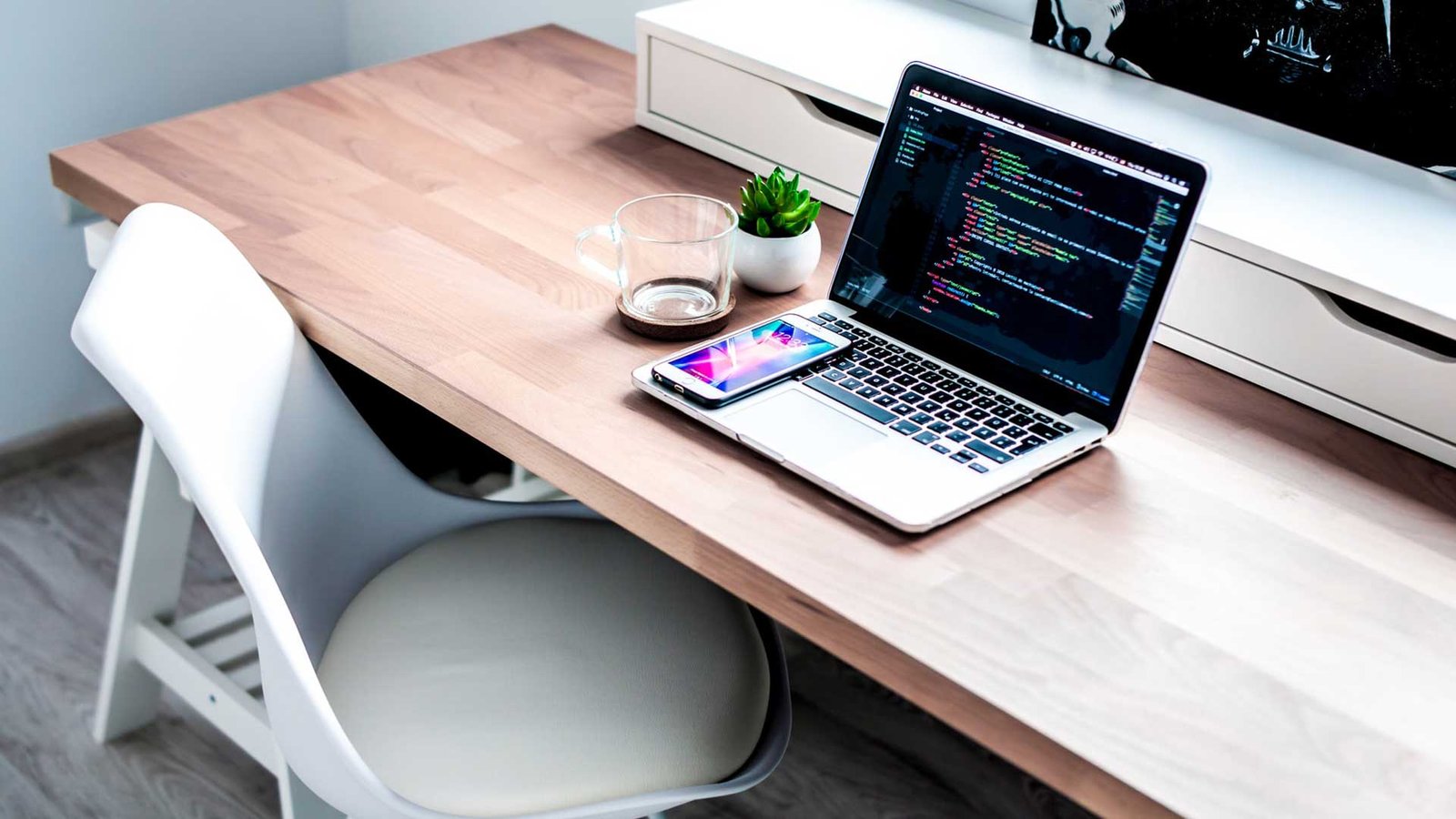