Table of Contents
PYTHON CONDITIONAL STATEMENTS
When there is no condition placed before any set of statements , the program will be executed in sequential manure. But when some condition is placed before a block of statements the flow of execution might change depends on the result evaluated by the condition. This type of statement is also called decision making statements or control statements. This type of statement may skip some set of statements based on the condition.
Logical Conditions Supported by Python
- Equal to (==) Eg: a == b
- Not Equal (!=)Eg : a != b
- Greater than (>) Eg: a > b
- Greater than or equal to (>=) Eg: a >= b
- Less than (<) Eg: a < b
- Less than or equal to (<=) Eg: a <= b
Indentation
C Program | Python |
x = 500; y = 200; if (x > y) { printf(“x is greater than y”); } else if(x == y) { printf(“x and y are equal”); } else { printf(“x is less than y”); } | x = 500 y = 200 if x > y: print(“x is greater than y”) elif x == y: print(“x and y are equal”) else: print(“x is less than y”) Indentation (At least one White Space instead of curly bracket) |
To represent a block of statements other programming languages like C, C++ uses “{ …}” curly – brackets , instead of this curly braces python uses indentation using white space which defines scope in the code. The example given below shows the difference between usage of Curly bracket and white space to represent a block of statement.
Without proper Indentation:
x = 500
y = 200
if x > y:
print(“x is greater than y”)
In the above example there is no proper indentation after if statement which will lead to Indentation error.
If Statement
The ‘if’ statement is written using “if” keyword, followed by a condition.If the condition is true the block will be executed. Otherwise, the control will be transferred to the firststatement after the block.
Syntax:
if<Boolean>:
<block>
In this statement, the order of execution is purely based on the evaluation of boolean expression.
Example:
x = 200
y = 100
if x > y:
print(“X is greater than Y”)
print(“End”)
Output :
X is greater than Y
End
In the above the value of x is greater than y , hence it executed the print statement whereas in the below example x is not greater than y hence it is not executed the first print statement
x = 100
y = 200
if x > y:
print(“X is greater than Y”)
print(“End”)
Output :
End
Elif
The elif keyword is useful for checking another condition when one condition is false.
Example
mark = 55
if (mark >=75):
print(“FIRST CLASS”)
elif mark >= 50:
print(“PASS”)
Output :
PASS
In the above the example, the first condition (mark >=75) is false then the control is transferred to the next condition (mark >=50), Thus, the keyword elif will be helpful for having more than one condition.
Else
The else keyword will be used as a default condition. i.e. When there are many conditions, whentheif-condition is not trueand all elif-conditionsare also not true, then else part will be executed..
Example :
mark = 10
if mark >= 75:
print(“FIRST CLASS”)
elif mark >= 50:
print(“PASS”)
else:
print(“FAIL”)
Output :
FAIL
In the example above, condition 1 and condition 2 fail. Noneof the preceding condition is true. Hence, the else part is executed.
You May Like to Browers More
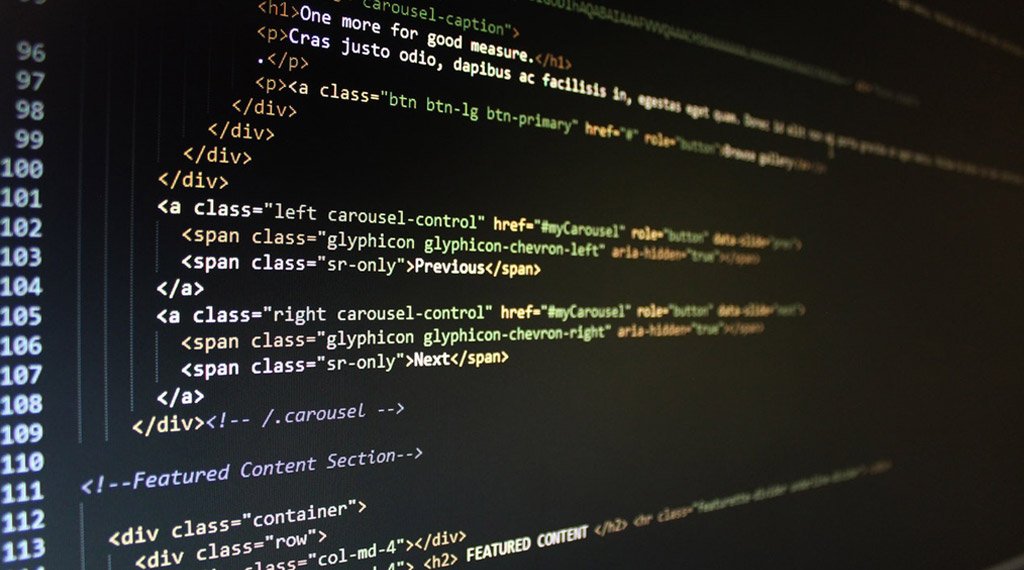
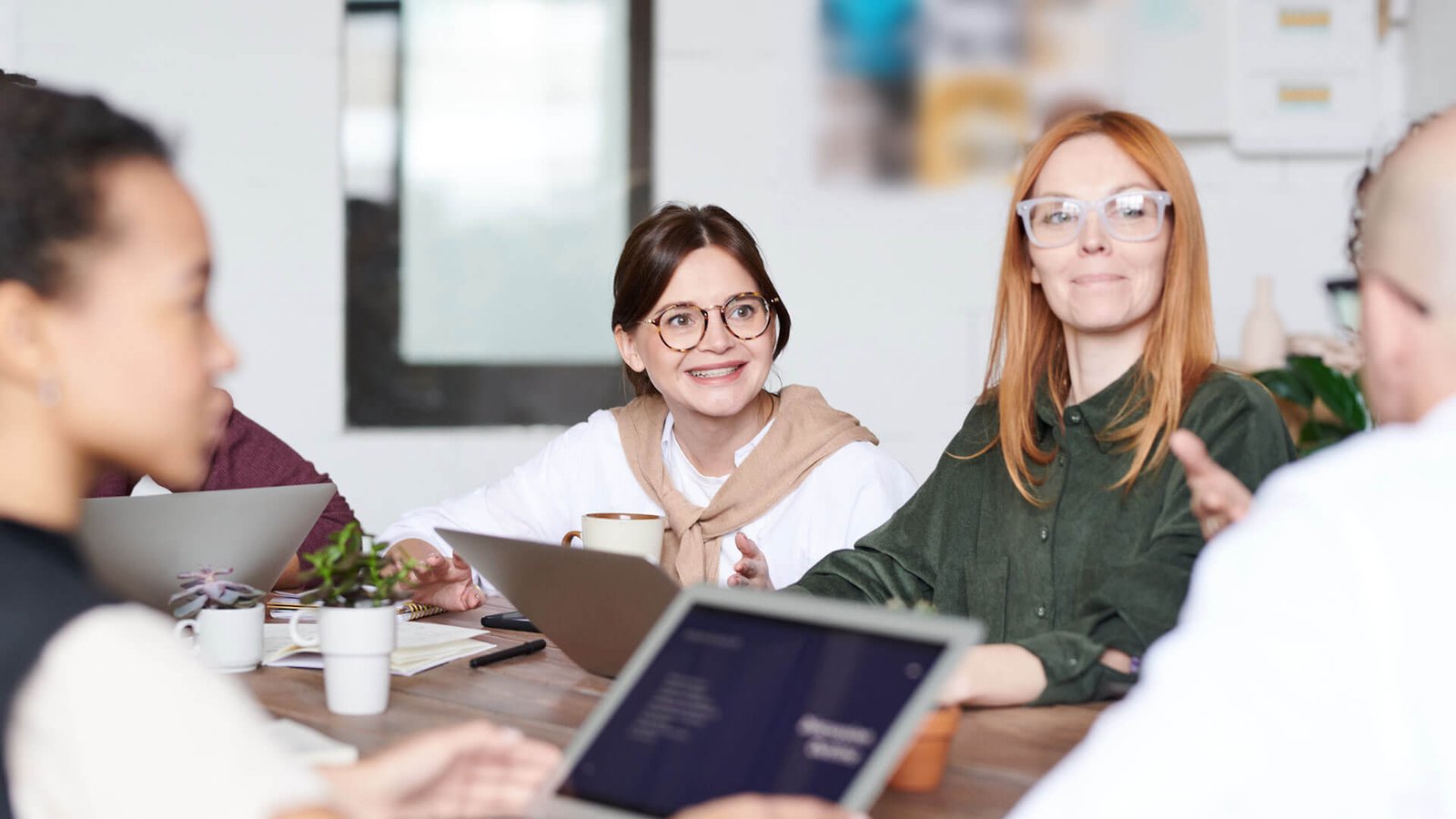
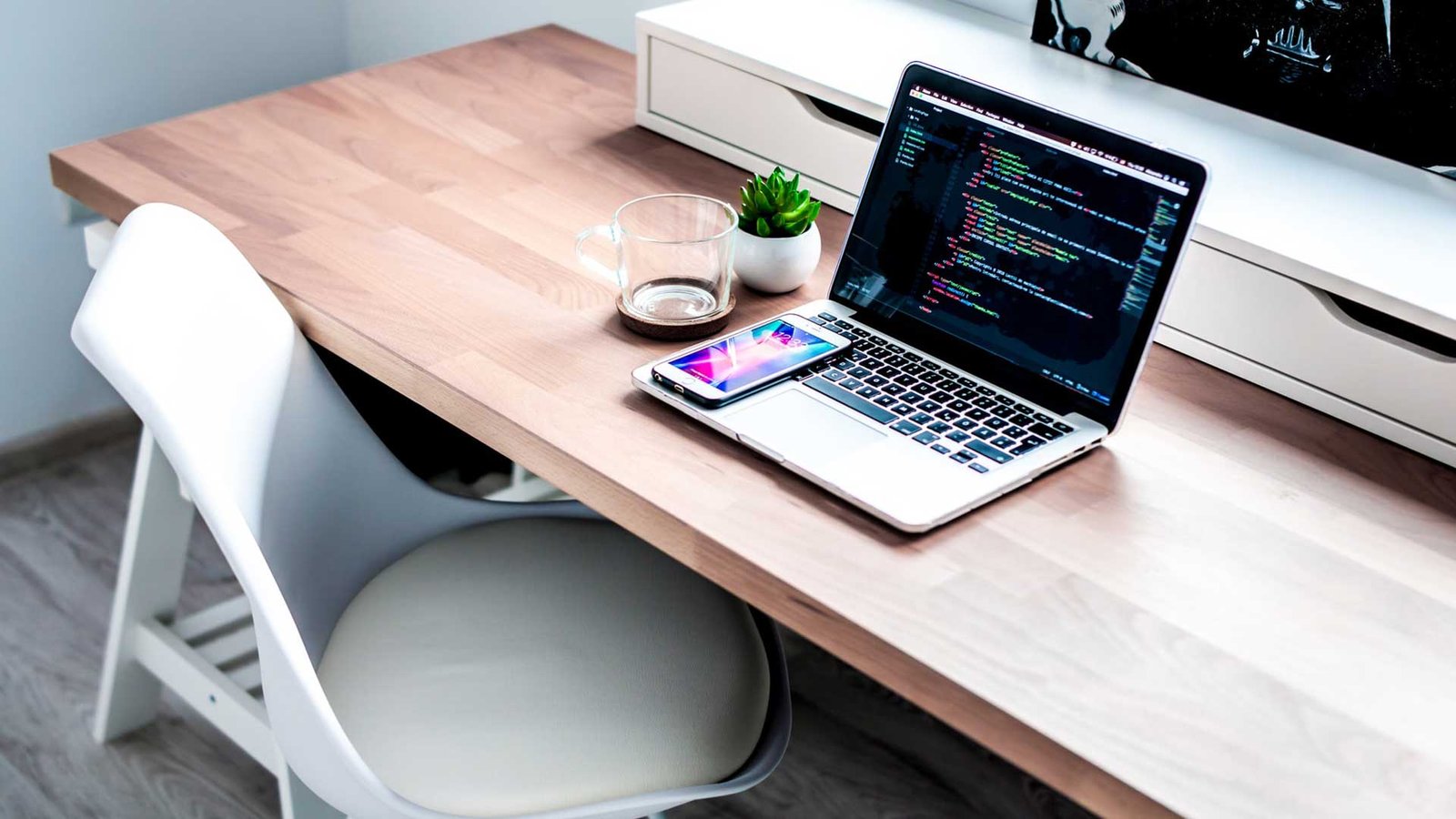